Folders
Folders provide a convenient mechanism for organizing your projects. Each folder may contain other folders and projects, and you copy and move their contents between folders.
Class Functions
byIdentifier(identifier: String) → (Folder or null) • Returns the Folder with the specified identifier, or null if no such folder exists.
Using the byIdentifier() method to locate a folder using the value of its primaryKey property (object ID):
Locating Folder by ID
Folder.byIdentifier('a0UDF6AROY8')
//--> [object Folder: Home Renovation]
Using the flattenedFolders property of the Database class and the byName(…) function of the SectionArray class to return an object reference to the first folder identified by name:
Locating 1st Instance of Folder by Name
fldr = flattenedFolders.byName('Home Renovation')
if(fldr){
console.log(fldr.id.primaryKey)
//--> "a0UDF6AROY8"
}
Instance Properties
Here are the properties of an instance of the Folder class:
after (Folder.ChildInsertionLocation r/o) • Returns a location refering to position just after this folder.
before (Folder.ChildInsertionLocation r/o) • Returns a location refering to position just before this folder.
beginning (Folder.ChildInsertionLocation r/o) • Returns a location refering to the beginning of the contained projects and folders in this folder.
ending (Folder.ChildInsertionLocation r/o) • Returns a location refering to the ending of the contained projects and folders in this folder.
children (Array of Project or Folder r/o) • Returns a sorted list of the folders and projects contained within this folder.
folders (Array of Folder r/o) • Returns the child folders of this folder.
flattenedChildren (SectionArray r/o) • An alias for flattenedSections.
flattenedFolders (FolderArray r/o) • Returns a flat array of all folders in this folder, sorted by their order in the database.
flattenedProjects (ProjectArray r/o) • Returns a flat array of all projects in this folder, sorted by their order in the database.
flattenedSections (SectionArray r/o) • Returns a flat array of all folders and project in this folder, sorted by their order in the database.
name (String) • The name of the folder.
parent (Folder or null r/o) • The parent Folder which contains this folder.
projects (Array of Project r/o) • Returns the projects contained directly as children of this folder.
sections (Array of Folder and/or Projects) • An Array containing Project and Folder objects. Works with the byName(…) function to return the first Project or Folder contained directly in this array with the given name.
status (Folder.Status) • The folder’s status.
Using the flattenedProjects property of the Folder class to iterate all projects within a specified folder:
Iterating All Projects in a Folder
folderName = 'Home Renovation'
fldr = flattenedFolders.byName(folderName)
if(fldr){
fldr.flattenedProjects.forEach(project => {
// process project
})
}
Folder.Status Class
The value for the status property of the Folder class:
Active (Folder.Status r/o) • The folder is active.
Dropped (Folder.Status r/o) • The folder has been dropped.
all (Array of Folder.Status r/o) • An array of all items of this enumeration.
Dropping a Folder
folder = folderNamed("Folder A")
if(folder){folder.status = Folder.Status.Dropped}
Creating Folders
New folders are created using the standard Javascript new item constructor, passing in values for the name and optinal insertion position parameters:
new Folder(name: String, position: Folder or Folder.ChildInsertionLocation or null) → (Folder) • Create a new directory.
Creating Folders within a Folder
fldr = new Folder("AAPL", library.beginning)
titles = ["1st Q","2nd Q","3rd Q","4th Q"]
titles.forEach(title => new Folder(title, fldr.ending))
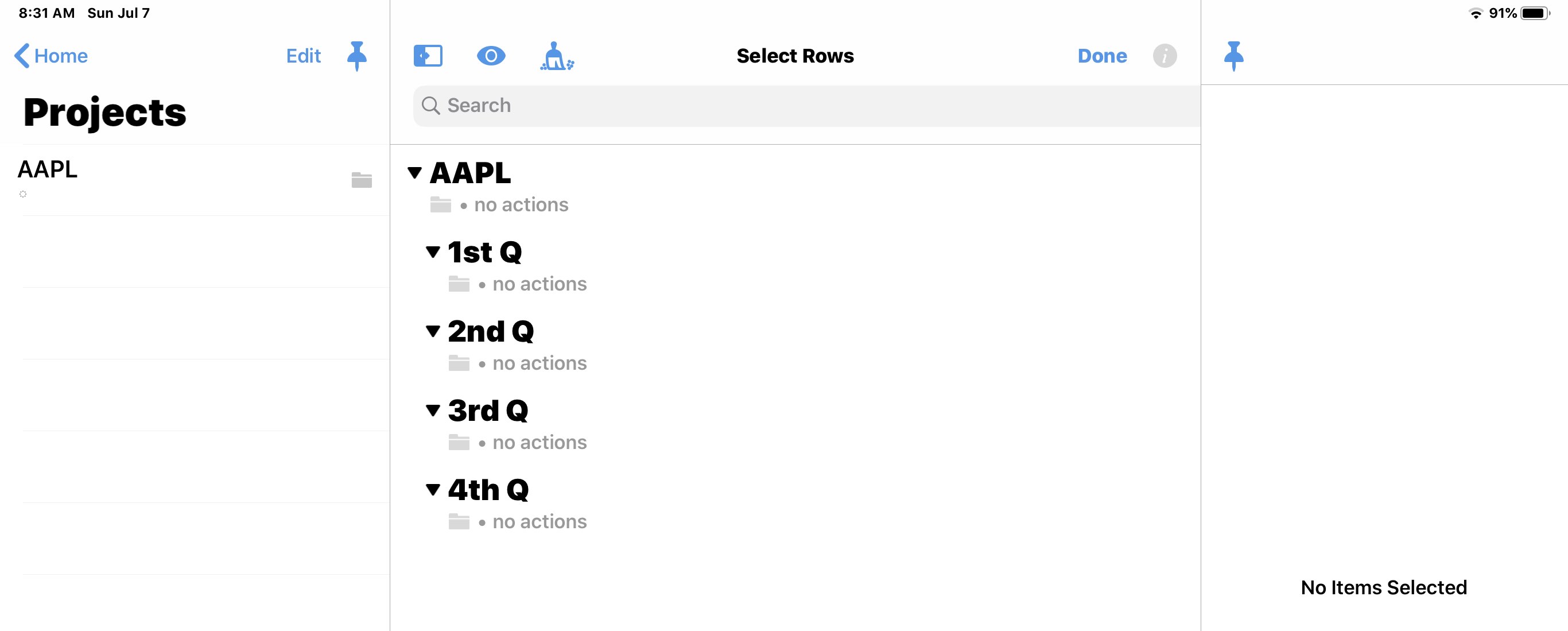
Creating a Folder Hierarchy
fldr = new Folder("Top Level Folder", library.beginning)
titles = ["1st Level","2nd Level","3rd Level","4th Level"]
titles.forEach(title => fldr = new Folder(title, fldr.beginning))
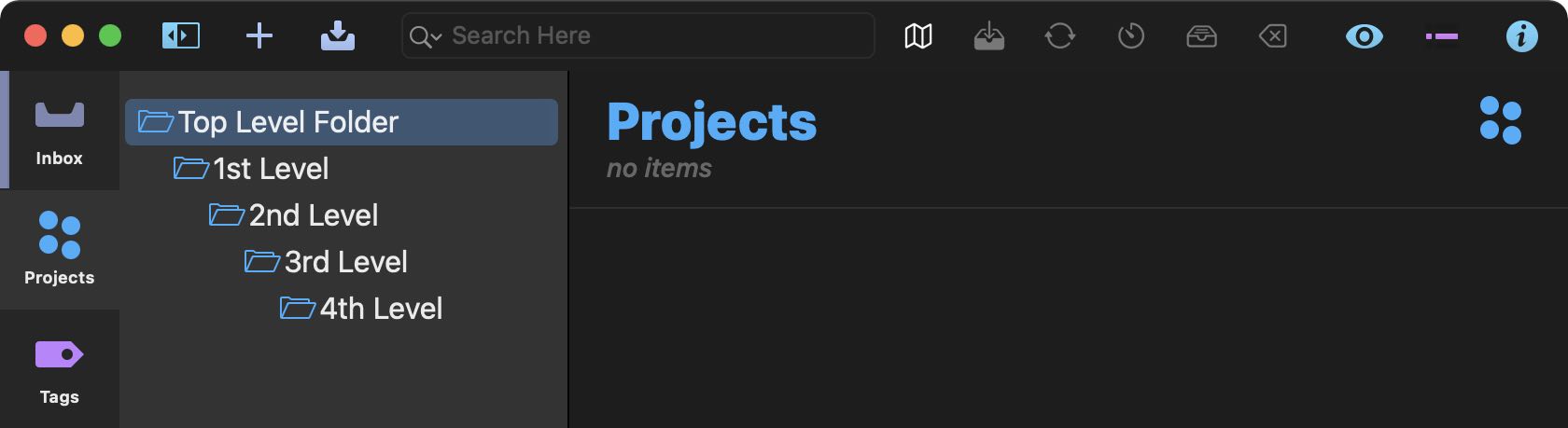
An example script for creating a new top-level folder for each month of the year:
Folder for Each Month
locale = "en-us"
monthNames = new Array()
for (i = 0; i < 12; i++) {
objDate = new Date()
objDate.setDate(1)
objDate.setMonth(i)
monthName = objDate.toLocaleString(locale, {month:"long"})
monthNames.push(monthName)
}
folderNames = folders.map(fldr => fldr.name)
monthNames.forEach(month => {
if(!folderNames.includes(month)){new Folder(month)}
})
Folder Instance Functions
Here are the functions that can be applied to instances of the Folder class:
folderNamed(name:String) → (Folder or null) • Returns the first child folder of this folder with the given name, or null.
projectNamed(name:String) → (Folder or null) • Returns the first child project of this folder with the given name, or null.
apply(function:Function) → (ApplyResult or null) • Calls the given function for this Folder and recursively into any child folders and projects. The tasks within any projects are not descended into.
For referencing specific folders within a folder hierarchy, use chained folderNamed() functions:
Folder Reference within a Folder Hierarchy
targetFolder = folderNamed("Top-Level").folderNamed("Secondary-Level").folderNamed("Third-Level")
Here’s a script that processes the net task of a specified project located within a specified top-level directory:
Next Task for Specified Folder Project
project = folderNamed("Fall Festival").projectNamed("Setup To-Do List")
if(project){
task = project.nextTask
// processing statements
}
NOTE: The Finding Items section contains examples of using global search functions like foldersMatching(…) to locate items specified by the values of their properties.
Folder Hierarchy
Folders may contain projects and other folders. Contained folders may also contain folders and projects, and so on.
Here's a macOS-only function that will return the path string to the selected library item (folder or project), for example:
Top-Level Folder > Secondary-Level Folder > Third-Level Folder > Selected Project
Hierarchy Path for Selected Folder or Project
function pathToSelectedLibraryItem(){
if (
document.windows[0].perspective === Perspective.BuiltIn.Projects &&
document.windows[0].sidebar.selectedNodes.length === 1
)
{
item = document.windows[0].sidebar.selectedNodes[0].object
pathItems = [item.name]
lvl = item.level
if(lvl > 1){
do {
item = item.parent
lvl = item.level
pathItems.unshift(item.object.name)
}
while (lvl > 1)
}
itemHeirarchy = pathItems.join(" > ")
return itemHeirarchy
}
return null
}
Sorting Folder Contents by Status
An example by Matt Johnson from the Omni Slack Channels demonstrating how to sort the projects of active folders by their status:
Sort Folder Projects by Status
activeFolders = flattenedFolders.filter(
folder => folder.status == Folder.Status.Active
)
activeFolders.forEach(fldr => {
projs = fldr.projects.sort().sort((a, b) => {
if (a.status < b.status) return 1;
if (a.status > b.status) return -1;
})
projs.forEach(project => {
moveSections([project], fldr.beginning)
})
})
Is a task within a specific folder?
Here’s a function that returns a Boolean (true or false) value indicating if a task is within a specified folder:
Is Task within Folder?
function hasAncestorFolder(task, folder){
parentProject = task.containingProject
if(parentProject === null){return false}
currentFolder = parentProject.parentFolder
while (currentFolder){
if (currentFolder === folder){
return true
}
currentFolder = currentFolder.parent
}
return false
};
IDs of all tasks within a folder
This example generates an array of IDs for all the tasks within all the active projects contained in the folder:
Get IDs of All Tasks within a Folder
folderName = 'Work'
fldr = flattenedFolders.byName(folderName)
if(fldr){
projs = fldr.flattenedProjects.filter(project => {
return project.status === Project.Status.Active
})
var taskIDs = new Array()
for (proj of projs){
IDs = proj.flattenedTasks.map(task => task.id.primaryKey)
taskIDs.push(IDs)
}
taskIDs = [].concat.apply([], taskIDs)
}