Plug-In: Append Mail Link to Note
Presents a form interface for entering the parameters used to create a mailto link (Recipient, Subject, CC, BCC, Body) that is appended to the note of the selected task. For the Body parameter “\n” will be replaced with a paragraph return. Provided values (except for the subject) will be retained and retrieved in subsequent uses.
Return to: OmniFocus Plug-In Collection
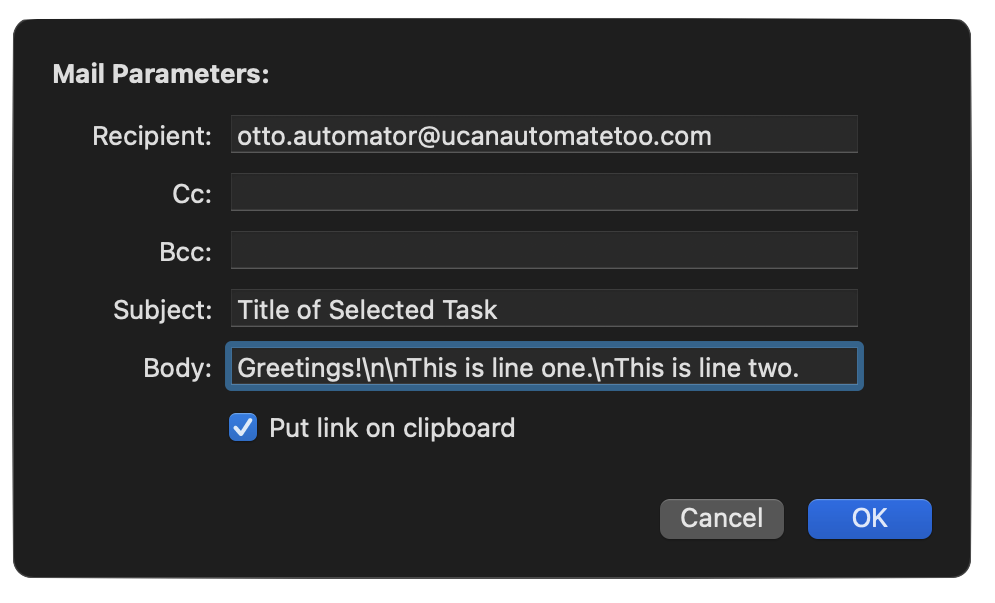
Append Mail Link to Note
/*{
"type": "action",
"targets": ["omnifocus"],
"author": "Otto Automator",
"identifier": "com.omni-automation.of.append-mail-link-to-note",
"version": "1.5",
"description": "Presents a form interface for entering the parameters used to create a mailto link (Recipient, Subject, CC, BCC, Body) that is appended to the note of the selected task. For the Body parameter “\n” will be replaced with a paragraph return.",
"label": "Append Mail Link to Note",
"shortLabel": "Add Mail Link",
"paletteLabel": "Add Mail Link",
"image": "envelope"
}*/
(() => {
var preferences = new Preferences() // NO ID = PLUG-IN ID
const action = new PlugIn.Action(async function(selection, sender){
// DEFAULTS
// NOTE: subject is disabled so that the value is the title of the selected task
var defaultLinkTitleValue = "(Mail Link)"
var defaultRecipientValue = null
var defaultCcValue = null
var defaultBccValue = null
//var defaultSubjectValue = null
var defaultBodyValue = null
var defaultShouldPlaceOnClipboard = false
var linkTitleValue = preferences.readString("linkTitleValue")
if(!linkTitleValue){linkTitleValue = defaultLinkTitleValue}
// RETRIEVE STORED FORM PARMATER VALUES
var recipientValue = preferences.readString("recipientValue")
if(!recipientValue){recipientValue = defaultRecipientValue}
var ccValue = preferences.readString("ccValue")
if(!ccValue){ccValue = defaultCcValue}
var bccValue = preferences.readString("bccValue")
if(!bccValue){bccValue = defaultBccValue}
//var subjectValue = preferences.readString("subjectValue")
//if(!subjectValue){subjectValue = defaultSubjectValue}
var bodyValue = preferences.readString("bodyValue")
if(!bodyValue){bodyValue = defaultBodyValue}
var shouldPlaceOnClipboardValue = preferences.readBoolean("shouldPlaceOnClipboardValue")
if(!shouldPlaceOnClipboardValue){shouldPlaceOnClipboardValue = defaultShouldPlaceOnClipboard}
// OBJECT REFERENCE FOR SELECTED PROJECT/TASK
var item = selection.databaseObjects[0]
// CREATE THE FORM AND ELEMENTS
var mailtoForm = new Form()
var linkTitleInput = new Form.Field.String("linkTitle", "Link Title", linkTitleValue)
var recipientsInput = new Form.Field.String("recipient", "Recipient", recipientValue)
var ccInput = new Form.Field.String("cc", "Cc", ccValue)
var bccInput = new Form.Field.String("bcc", "Bcc", bccValue)
var subjectInput = new Form.Field.String("subject", "Subject", item.name)
var bodyInput = new Form.Field.String("body", "Body", bodyValue)
var clipboardInput = new Form.Field.Checkbox(
"shouldReplaceClipboard",
"Put link on clipboard",
shouldPlaceOnClipboardValue
)
mailtoForm.addField(linkTitleInput)
mailtoForm.addField(recipientsInput)
mailtoForm.addField(ccInput)
mailtoForm.addField(bccInput)
mailtoForm.addField(subjectInput)
mailtoForm.addField(bodyInput)
mailtoForm.addField(clipboardInput)
mailtoForm.validate = function(formObject){
var recipient = formObject.values['recipient']
var cc = formObject.values['cc']
var bcc = formObject.values['bcc']
if(recipient){
if (/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/.test(recipient)){
recipientStatus = true
} else {
throw "ERROR: not a valid recipient address."
}
} else {throw "NOTE: A recipient address is required."}
if(cc){
if (/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/.test(cc)){
ccStatus = true
} else {
throw "ERROR: not a valid Cc address."
}
}
if(bcc){
if (/^\w+([\.-]?\w+)*@\w+([\.-]?\w+)*(\.\w{2,3})+$/.test(bcc)){
bccStatus = true
} else {
throw "ERROR: not a valid Bcc address."
}
}
return true
}
var formPrompt = "Mail Parameters:"
var buttonTitle = "OK"
var formObject = await mailtoForm.show(formPrompt, buttonTitle)
// RETRIEVE VALUES
var linkTitle = formObject.values['linkTitle']
var recipient = formObject.values['recipient']
var cc = formObject.values['cc']
var bcc = formObject.values['bcc']
var subject = formObject.values['subject']
var body = formObject.values['body']
var shouldReplaceClipboard = formObject.values['shouldReplaceClipboard']
// CONSRUCT MAILTO: URL
var args = [];
if (cc){
args.push('cc=' + cc);
}
if (bcc){
args.push('bcc=' + bcc);
}
if (subject){
args.push('subject=' + encodeURIComponent(subject));
}
if (body){
args.push('body=' + encodeURIComponent(body).replaceAll("%5Cn","%0A"))
}
var url = 'mailto:' + recipient
if (args.length > 0){
url += '?' + args.join('&')
}
// CREATE THE LINK OBJECT
linkURL = URL.fromString(url)
linkTitle = (linkTitle) ? linkTitle:defaultLinkTitleValue
// APPEND TO NOTE
if(app.userVersion.versionString.startsWith("4")){
noteObj = item.noteText
linkObj = new Text(linkTitle, noteObj.style)
newLineObj = new Text("\n", noteObj.style)
style = linkObj.styleForRange(linkObj.range)
style.set(Style.Attribute.Link, linkURL)
if (noteObj.string.length > 0){noteObj.append(newLineObj)}
noteObj.append(linkObj)
node = document.windows[0].content.selectedNodes[0]
node.expandNote(false)
} else {
if (task.note.length === 0){
task.appendStringToNote(url)
} else {
task.appendStringToNote(`\n${url}`)
}
}
// PLACE ON CLIPBOARD
if(shouldReplaceClipboard){Pasteboard.general.string = url}
// STORE THE NEW VALUES
preferences.write("linkTitleValue", linkTitle)
preferences.write("recipientValue", recipient)
preferences.write("ccValue", cc)
preferences.write("bccValue", bcc)
preferences.write("bodyValue", body)
preferences.write("shouldPlaceOnClipboardValue", shouldReplaceClipboard)
});
action.validate = function(selection, sender){
items = selection.databaseObjects.filter(
item => (item instanceof Project || item instanceof Task)
)
return (items.length === 1)
};
return action;
})();